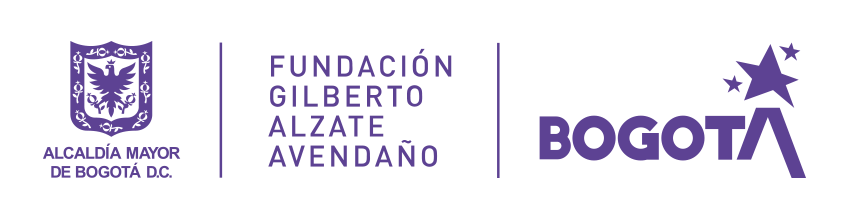
http://example.com/foo/bar
)'),
'proto-rel' => t('Protocol relative URL (//example.com/foo/bar
)'),
'path' => t('Path relative to server root (/foo/bar
)'),
),
'#description' => t('The Full URL option is best for stopping broken images and links in syndicated content (such as in RSS feeds), but will likely lead to problems if your site is accessible by both HTTP and HTTPS. Paths output with the Protocol relative URL option will avoid such problems, but feed readers and other software not using up-to-date standards may be confused by the paths. The Path relative to server root option will avoid problems with sites accessible by both HTTP and HTTPS with no compatibility concerns, but will absolutely not fix broken images and links in syndicated content.'),
'#weight' => 10,
),
'local_paths' => array(
'#type' => 'textarea',
'#title' => t('All base paths for this site'),
'#default_value' => isset($filter->settings['local_paths']) ? $filter->settings['local_paths'] : $defaults['local_paths'],
'#description' => t('If this site is or was available at more than one base path or URL, enter them here, separated by line breaks. For example, if this site is live at http://example.com/
but has a staging version at http://dev.example.org/staging/
, you would enter both those URLs here. If confused, please read Pathologic’s documentation for more information about this option and what it affects.', array('!docs' => 'http://drupal.org/node/257026')),
'#weight' => 20,
),
);
}
/**
* Pathologic filter callback.
*
* Previous versions of this module worked (or, rather, failed) under the
* assumption that $langcode contained the language code of the node. Sadly,
* this isn't the case.
* @see http://drupal.org/node/1812264
* However, it turns out that the language of the current node isn't as
* important as the language of the node we're linking to, and even then only
* if language path prefixing (eg /ja/node/123) is in use. REMEMBER THIS IN THE
* FUTURE, ALBRIGHT.
*
* The below code uses the @ operator before parse_url() calls because in PHP
* 5.3.2 and earlier, parse_url() causes a warning of parsing fails. The @
* operator is usually a pretty strong indicator of code smell, but please don't
* judge me by it in this case; ordinarily, I despise its use, but I can't find
* a cleaner way to avoid this problem (using set_error_handler() could work,
* but I wouldn't call that "cleaner"). Fortunately, Drupal 8 will require at
* least PHP 5.3.5, so this mess doesn't have to spread into the D8 branch of
* Pathologic.
* @see https://drupal.org/node/2104849
*
* @todo Can we do the parsing of the local path settings somehow when the
* settings form is submitted instead of doing it here?
*/
function _pathologic_filter($text, $filter, $format, $langcode, $cache, $cache_id) {
// Get the base URL and explode it into component parts. We add these parts
// to the exploded local paths settings later.
global $base_url;
$base_url_parts = @parse_url($base_url . '/');
// Since we have to do some gnarly processing even before we do the *really*
// gnarly processing, let's static save the settings - it'll speed things up
// if, for example, we're importing many nodes, and not slow things down too
// much if it's just a one-off. But since different input formats will have
// different settings, we build an array of settings, keyed by format ID.
$cached_settings = &drupal_static(__FUNCTION__, array());
if (!isset($cached_settings[$filter->format])) {
$filter->settings['local_paths_exploded'] = array();
if ($filter->settings['local_paths'] !== '') {
// Build an array of the exploded local paths for this format's settings.
// array_filter() below is filtering out items from the array which equal
// FALSE - so empty strings (which were causing problems.
// @see http://drupal.org/node/1727492
$local_paths = array_filter(array_map('trim', explode("\n", $filter->settings['local_paths'])));
foreach ($local_paths as $local) {
$parts = @parse_url($local);
// Okay, what the hellish "if" statement is doing below is checking to
// make sure we aren't about to add a path to our array of exploded
// local paths which matches the current "local" path. We consider it
// not a match, if…
// @todo: This is pretty horrible. Can this be simplified?
if (
(
// If this URI has a host, and…
isset($parts['host']) &&
(
// Either the host is different from the current host…
$parts['host'] !== $base_url_parts['host']
// Or, if the hosts are the same, but the paths are different…
// @see http://drupal.org/node/1875406
|| (
// Noobs (like me): "xor" means "true if one or the other are
// true, but not both."
(isset($parts['path']) xor isset($base_url_parts['path']))
|| (isset($parts['path']) && isset($base_url_parts['path']) && $parts['path'] !== $base_url_parts['path'])
)
)
) ||
// Or…
(
// The URI doesn't have a host…
!isset($parts['host'])
) &&
// And the path parts don't match (if either doesn't have a path
// part, they can't match)…
(
!isset($parts['path']) ||
!isset($base_url_parts['path']) ||
$parts['path'] !== $base_url_parts['path']
)
) {
// Add it to the list.
$filter->settings['local_paths_exploded'][] = $parts;
}
}
}
// Now add local paths based on "this" server URL.
$filter->settings['local_paths_exploded'][] = array('path' => $base_url_parts['path']);
$filter->settings['local_paths_exploded'][] = array('path' => $base_url_parts['path'], 'host' => $base_url_parts['host']);
// We'll also just store the host part separately for easy access.
$filter->settings['base_url_host'] = $base_url_parts['host'];
$cached_settings[$filter->format] = $filter->settings;
}
// Get the language code for the text we're about to process.
$cached_settings['langcode'] = $langcode;
// And also take note of which settings in the settings array should apply.
$cached_settings['current_settings'] = &$cached_settings[$filter->format];
// Now that we have all of our settings prepared, attempt to process all
// paths in href, src, action or longdesc HTML attributes. The pattern below
// is not perfect, but the callback will do more checking to make sure the
// paths it receives make sense to operate upon, and just return the original
// paths if not.
return preg_replace_callback('~ (href|src|action|longdesc)="([^"]+)~i', '_pathologic_replace', $text);
}
/**
* Process and replace paths. preg_replace_callback() callback.
*/
function _pathologic_replace($matches) {
// Get the base path.
global $base_path;
// Get the settings for the filter. Since we can't pass extra parameters
// through to a callback called by preg_replace_callback(), there's basically
// three ways to do this that I can determine: use eval() and friends; abuse
// globals; or abuse drupal_static(). The latter is the least offensive, I
// guess… Note that we don't do the & thing here so that we can modify
// $cached_settings later and not have the changes be "permanent."
$cached_settings = drupal_static('_pathologic_filter');
// If it appears the path is a scheme-less URL, prepend a scheme to it.
// parse_url() cannot properly parse scheme-less URLs. Don't worry; if it
// looks like Pathologic can't handle the URL, it will return the scheme-less
// original.
// @see https://drupal.org/node/1617944
// @see https://drupal.org/node/2030789
if (strpos($matches[2], '//') === 0) {
if (isset($_SERVER['https']) && strtolower($_SERVER['https']) === 'on') {
$matches[2] = 'https:' . $matches[2];
}
else {
$matches[2] = 'http:' . $matches[2];
}
}
// Now parse the URL after reverting HTML character encoding.
// @see http://drupal.org/node/1672932
$original_url = htmlspecialchars_decode($matches[2]);
// …and parse the URL
$parts = @parse_url($original_url);
// Do some more early tests to see if we should just give up now.
if (
// If parse_url() failed, give up.
$parts === FALSE
|| (
// If there's a scheme part and it doesn't look useful, bail out.
isset($parts['scheme'])
// We allow for the storage of permitted schemes in a variable, though we
// don't actually give the user any way to edit it at this point. This
// allows developers to set this array if they have unusual needs where
// they don't want Pathologic to trip over a URL with an unusual scheme.
// @see http://drupal.org/node/1834308
// "files" and "internal" are for Path Filter compatibility.
&& !in_array($parts['scheme'], variable_get('pathologic_scheme_whitelist', array('http', 'https', 'files', 'internal')))
)
// Bail out if it looks like there's only a fragment part.
|| (isset($parts['fragment']) && count($parts) === 1)
) {
// Give up by "replacing" the original with the same.
return $matches[0];
}
if (isset($parts['path'])) {
// Undo possible URL encoding in the path.
// @see http://drupal.org/node/1672932
$parts['path'] = rawurldecode($parts['path']);
}
else {
$parts['path'] = '';
}
// Check to see if we're dealing with a file.
// @todo Should we still try to do path correction on these files too?
if (isset($parts['scheme']) && $parts['scheme'] === 'files') {
// Path Filter "files:" support. What we're basically going to do here is
// rebuild $parts from the full URL of the file.
$new_parts = @parse_url(file_create_url(file_default_scheme() . '://' . $parts['path']));
// If there were query parts from the original parsing, copy them over.
if (!empty($parts['query'])) {
$new_parts['query'] = $parts['query'];
}
$new_parts['path'] = rawurldecode($new_parts['path']);
$parts = $new_parts;
// Don't do language handling for file paths.
$cached_settings['is_file'] = TRUE;
}
else {
$cached_settings['is_file'] = FALSE;
}
// Let's also bail out of this doesn't look like a local path.
$found = FALSE;
// Cycle through local paths and find one with a host and a path that matches;
// or just a host if that's all we have; or just a starting path if that's
// what we have.
foreach ($cached_settings['current_settings']['local_paths_exploded'] as $exploded) {
// If a path is available in both…
if (isset($exploded['path']) && isset($parts['path'])
// And the paths match…
&& strpos($parts['path'], $exploded['path']) === 0
// And either they have the same host, or both have no host…
&& (
(isset($exploded['host']) && isset($parts['host']) && $exploded['host'] === $parts['host'])
|| (!isset($exploded['host']) && !isset($parts['host']))
)
) {
// Remove the shared path from the path. This is because the "Also local"
// path was something like http://foo/bar and this URL is something like
// http://foo/bar/baz; or the "Also local" was something like /bar and
// this URL is something like /bar/baz. And we only care about the /baz
// part.
$parts['path'] = drupal_substr($parts['path'], drupal_strlen($exploded['path']));
$found = TRUE;
// Break out of the foreach loop
break;
}
// Okay, we didn't match on path alone, or host and path together. Can we
// match on just host? Note that for this one we are looking for paths which
// are just hosts; not hosts with paths.
elseif ((isset($parts['host']) && !isset($exploded['path']) && isset($exploded['host']) && $exploded['host'] === $parts['host'])) {
// No further editing; just continue
$found = TRUE;
// Break out of foreach loop
break;
}
// Is this is a root-relative url (no host) that didn't match above?
// Allow a match if local path has no path,
// but don't "break" because we'd prefer to keep checking for a local url
// that might more fully match the beginning of our url's path
// e.g.: if our url is /foo/bar we'll mark this as a match for
// http://example.com but want to keep searching and would prefer a match
// to http://example.com/foo if that's configured as a local path
elseif (!isset($parts['host']) && (!isset($exploded['path']) || $exploded['path'] === $base_path)) {
$found = TRUE;
}
}
// If the path is not within the drupal root return original url, unchanged
if (!$found) {
return $matches[0];
}
// Okay, format the URL.
// If there's still a slash lingering at the start of the path, chop it off.
$parts['path'] = ltrim($parts['path'],'/');
// Examine the query part of the URL. Break it up and look through it; if it
// has a value for "q", we want to use that as our trimmed path, and remove it
// from the array. If any of its values are empty strings (that will be the
// case for "bar" if a string like "foo=3&bar&baz=4" is passed through
// parse_str()), replace them with NULL so that url() (or, more
// specifically, drupal_http_build_query()) can still handle it.
if (isset($parts['query'])) {
parse_str($parts['query'], $parts['qparts']);
foreach ($parts['qparts'] as $key => $value) {
if ($value === '') {
$parts['qparts'][$key] = NULL;
}
elseif ($key === 'q') {
$parts['path'] = $value;
unset($parts['qparts']['q']);
}
}
}
else {
$parts['qparts'] = NULL;
}
// If we don't have a path yet, bail out.
if (!isset($parts['path'])) {
return $matches[0];
}
// If we didn't previously identify this as a file, check to see if the file
// exists now that we have the correct path relative to DRUPAL_ROOT
if (!$cached_settings['is_file']) {
$cached_settings['is_file'] = !empty($parts['path']) && is_file(DRUPAL_ROOT . '/'. $parts['path']);
}
// Okay, deal with language stuff.
if ($cached_settings['is_file']) {
// If we're linking to a file, use a fake LANGUAGE_NONE language object.
// Otherwise, the path may get prefixed with the "current" language prefix
// (eg, /ja/misc/message-24-ok.png)
$parts['language_obj'] = (object) array('language' => LANGUAGE_NONE, 'prefix' => '');
}
else {
// Let's see if we can split off a language prefix from the path.
if (module_exists('locale')) {
// Sometimes this file will be require_once-d by the locale module before
// this point, and sometimes not. We require_once it ourselves to be sure.
require_once DRUPAL_ROOT . '/includes/language.inc';
list($language_obj, $path) = language_url_split_prefix($parts['path'], language_list());
if ($language_obj) {
$parts['path'] = $path;
$parts['language_obj'] = $language_obj;
}
}
}
// If we get to this point and $parts['path'] is now an empty string (which
// will be the case if the path was originally just "/"), then we
// want to link to A propósito de Conversaciones Cruzadas sobre arte urbano, la FUGA habló con los artistas Yohn Smith y Roberto Peremese. Conozca su trayectoria y el impacto de sus creaciones en los Centros de ciudades como Bogotá y Lima.
Smith: la memoria de los muros del Centro de Bogotá
Yhon Smith Sierra se mueve como pez en el agua en el centro. El respeto que ha ganado en el circuito callejero como grafitero y con su grupo de rap Todo Copas, le ha permitido llegar a muros prohibidos para muchos. Precisamente, su conocimiento del centro fue su tiquete para el espacio virtual “Conversaciones cruzadas sobre arte urbano”, que cuenta con el apoyo de la Secretaría de Cultura Recreación y Deporte, a través de su estrategia Conversaciones Cruzadas, y la Fundación Gilberto Alzate Avendaño (FUGA).
Smith se involucró en la cultura del grafiti a finales de los años 90, cuando hacía parte de un grupo musical de hip hop. En el 2007 creó la agrupación Todo Copas y en el mismo año realizó el Festival de Arte Callejero en “La L” o antigua calle del Bronx, que se extendió hasta el 2015. Ha colaborado en la reconstrucción de la memoria local y cultural de lo que fue el Bronx para el Co-Laboratorio de Creación y Memoria “La Esquina Redonda”, un espacio que hará parte del Proyecto Bronx Distrito Creativo que lidera la Alcaldía Mayor de Bogotá por medio de la FUGA. En la actualidad trabaja en el sexto álbum musical con su agrupación y continúa con el grafiti a través de la técnica de aerografía textil.
Como él mismo dice, se volvió un científico y vive de lo que se inventa. Pinta camisas, gorras, tenis y recibe regalías de Youtube. Además participa en cuanto festival de rap hay en Bogotá, tanto como interprete como en las carpas de emprendimiento, en donde deja huella con sus aerografías. Además, conoce la movida del grafiti en el centro de la ciudad.
¿Cómo fue su evolución como artista?
Parchando con unos pelados de Las Cruces fue que conocí el grafiti. Estaba la moda del rap y en furor la ropa ancha y toda esa cuestión. En el 97 tuve mi grupo, que todavía está vigente, se llama Engendros del pantano. En ese tiempo el rap estaba ligado al grafiti y yo era el grafitero del grupo. Luego tuve la oportunidad de participar en el proyecto Jóvenes conviven por Bogotá y ahí conocí un aerógrafo, especialicé mi dibujo y lo pasé a camisetas. Como tenía reconocimiento por el grupo empecé a hacer grafitis con el nombre del grupo y eso me abrió las puertas del emprendimiento.
¿Cuáles son las temáticas que aborda?
Es un estilo arraigado a mi grupo actual, Todo Copas. Lo que hago se centra en un personaje que es un calvo, que saqué de mis facciones, y lo dibujo en diferentes situaciones: haciendo grafitis, cantando, con un tornamesa, a veces como un bandido con armas; básicamente es dibujar mi calvo siempre. Eso ha hecho que la gente de los alrededores me pida que les haga dibujos para sus negocios. Es otra faceta. Me salen bastante para locales de tatuajes, barberías y he aerografiado tiendas donde venden artículos de hip hop.
¿Cómo empezó su relación con el centro?
Cuando inicié con Engendros del pantano, en el 97, todavía estaba El Cartucho. Como parchaba en el gueto muchos habitantes de calle conocían de mi trabajo y eso me permitía llegar a puntos donde había cambuches y me era fácil pintar. No corría riesgos de seguridad, nos distinguían. Por tener esos contactos tenía pista para pintar. En ese sector conocí muchos de los personajes que tuvieron el monopolio en Calle del Bronx. Cuando se acabó el cartucho esas firmas se fueron para la calle del Bronx y allá siguió el parche, y pude seguir manifestándome con el dibujo en esos sectores.
Con su agrupación Todo Copas usted organizó el Festival Callejero del Bronx durante varios años. Háblenos de eso
Estaba dirigido a los que iban a parchar allá. En ese festival las manifestaciones eran de los habitantes de ahí y no todo era rap. Había habitantes de calle que cantaban rancheras, vallenatos y declamaban poesía, además a la olla iban los que cantaban en los buses. También estaban los de los malabares. Llevábamos tarima, buen sonido y las pistas. Era como el festival local de cualquier localidad pero solo para habitantes de calle.
¿Aparte de las presentaciones artísticas hacían algo de grafiti en ese espacio?
No había puertas abiertas para los grafiteros. Solo nos dejaban rayar a nosotros, que éramos de Todo Copas. Pintábamos donde fuera: en las divisiones de los locales, dentro de los rockolas, en las maquinitas, en esos cuartuchos por allá adentro, en las paredes esquineadas. Tuve muchísimos grafitis ahí. Con Todo Copas hicimos allá más de un video. Hay uno que se llama Matices del pantano, que en una toma se ve un dibujo donde estaba el basurero. Tuve que pedir un andamio prestado, es un dibujo alto, visible, que tenía mi nombre, Smith y un diablito rojo. Ese era uno de los que más veía la gente, estaba encima de la puerta y parecía un aviso.
“La libertad que me ofrece el grafiti era y es infinita”: Roberto Peremese
Artista plástico, ilustrador, escritor y muralista peruano, Roberto Peremese ha recorrido el mundo con su arte. En compañía del colombiano Yhon Smith Sierra participó en “Conversaciones cruzadas sobre arte urbano”, un espacio que se realiza en el marco de la IV edición de la “Semana del Perú” en Colombia, organizada por la Embajada del Perú en nuestro país, con el apoyo de la Secretaría de Cultura Recreación y Deporte y la Fundación Gilberto Alzate Avendaño (FUGA).
- En su portafolio anuncia “psicodelia andina, cultura ancestral, folklore urbano y rap”. Explíquenos este concepto con el que define su estilo.
Mi camino artístico es campo abierto, es decir, no persigo ni deseo una autodefinición. Si bien tengo intereses y tendencias, estas seguirán transformándose con el encuentro de otros. La psicodelia es la manifestación del alma, considero que es un esfuerzo por proyectar mi mundo interior través de los colores, y formas, esto se debe a mi acercamiento al paisaje serrano peruano, su naturaleza e indumentaria. El conocimiento ancestral surge través de la cosmovisión andina, el mundo nahual y el chamanismo amazónico tras vivir un tiempo aprendiendo pintura en la selva. Mi quehacer en torno a la calle y su folklore va desde de mi barrio, colectivos y tribus urbanas con tradiciones que aparecen, se mantienen, mutan y desaparecen. En un principio fue nuestra apropiación con el hip hop y luego frecuentar, afrontar y abrazar la diversidad en mi cotidiano en los siguientes años.
¿Por qué se inclinó por el grafiti y el arte urbano?
El grafiti llegó antes que toda inclinación. La libertad que me ofrece el grafiti era y es infinita, a pesar de que tenga sus propios códigos y lenguaje, considero que es una fuente inagotable de procesos creativos en deconstrucción.
El grafiti ha estado siempre ligado el hip hop, a lo callejero e incluso a lo marginal. Pero en los últimos años prestigiosas marcas han recurrido a esta expresión como mecanismo de promoción. ¿Está evolucionando el grafiti o está perdiendo su esencia?
Particularmente, el grafiti que realizo no lo quieren las empresas, jaajaja, mejor así. Si mi trabajo coincide con el mensaje de la marca, se abre un diálogo de colaboración. Es muy interesante preguntar cómo vive y cómo ha vivido el artista urbano. La esencia del grafiti es de naturaleza ilegal. Otra forma es el arte urbano/street art, que al ser institucionalizado poco a poco, es aceptado y consumido, con posibilidades y limitaciones. En general la evolución del arte en su éxtasis y decadencia no creo que tenga que ver con la empresa, sino con la labor y entrega del artista a su quehacer creativo que lo legitima como ser humano. Me gusta la involución, he aprendido mucho más en ese sentido.
- Háblenos de su relación con el Centro de Lima y los trabajos que ha hecho allí.
Desde los 18 años tengo un vínculo fuerte con el centro histórico; en medio de calles con olor a orín, vagabundos, contracultura, comida variada a costos pequeños y manifestaciones contra el gobierno eran las postales de cada día. Estudiaba artes plásticas y se idealizaba la bohemia, un romanticismo decadente y delirante de una burbuja de párvulos y me meto en el saco. Desarrollé algunos grafitis y algunos murales cerca de la Escuela Nacional de Bellas Artes, ambos borrados por la alcaldía de turno. Lo interesante es la reacción que generaba en la calle con diálogos, feedback, recomendaciones, preguntas y finalmente la contemplación final en medio del ruido capitalista contaminante, muy contrario a la realidad en la escuela, tras enjaular a los artistas y sus obras con un halo de super ego. Hoy la ciudad está más atendida, y se han podido gestar murales, que poco a poco van teniendo más espacios de conservación.
- ¿Tiene el grafiti en Perú apoyo del gobierno (nacional y local) para su desarrollo?
Los espacios para festivales y encuentros, no solo de grafiti, sino también de arte urbano y hip hop, han sido iniciativa del artista-gestor, siendo la autogestión clave para el desarrollo del colectivo y la comunidad. Si bien el apoyo a través del tiempo ha ido de menos a más, hoy en día es posible hablar de incentivos del ministerio para proyectos personales o colectivos en relación con el grafiti y arte urbano.
-¿Cuántas veces ha visitado Colombia y qué trabajos ha hecho?
Cuatro o cinco, no recuerdo bien. He sido invitado en todas las ocasiones, primero a participar en Hip Hop al Parque del 2011, luego al festival Sur Fest por dos años consecutivos y otro por un concurso ganador dentro de los incentivos en Bogotá.
-¿Qué le falta al grafiti para que sea un movimiento global?
Ya lo es.