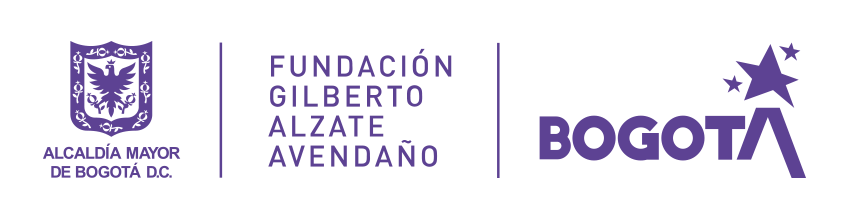
Bogotá, 14 de abril de 2021. A partir del 14 de abril y hasta el 14 de julio se abre a la convocatoria nacional del VII Salón BAT de Arte Popular, Colombia y el Medio Ambiente. Dirigida a los artistas empíricos para que hagan un llamado sobre la responsabilidad que todos tenemos en el cuidado y la preservación del medio ambiente.
El Salón BAT de Arte Popular es un espacio de reconocimiento del arte popular en Colombia que promueve el emprendimiento, fortalece la interacción del público con el arte empírico a través de exposiciones, actividades académicas y pedagógicas, producciones audiovisuales y actividades en plataformas virtuales que fomentan la creatividad y propician la comercialización del arte popular, contribuyendo al desarrollo social y económico del país.
Es la continuación de un proyecto que se inició en el año 2004 y que a lo largo de sus seis ediciones se ha constituido como una iniciativa con impacto nacional sobre este género de artes plásticas en nuestro país, así como una plataforma de cobertura nacional, donde los artistas y sus obras se presentan como interpretación de las dinámicas socioculturales de las regiones del país.
El lanzamiento de la convocatoria nacional se realiza con de la Gobernación de Bolívar, el Instituto de Cultura y Turismo de Bolívar ICULTUR, para hacerle un homenaje al artista magangueleño Eduardo Butrón Hodwalker e invitar a los artistas de todos los territorios de Colombia a que participen.
En su séptima versión el Salón BAT de Arte Popular cuenta con el apoyo del Ministerio de Cultura, programa Nacional de Concertación Cultural; el Gobierno de Colombia con los Programas de Desarrollo con Enfoque Territorial PDET, el Ministerio de Comercio, Industria y Turismo, con el Fondo Nacional del Turismo FONTUR; el Instituto Nacional Penitenciario y Carcelario INPEC, el Museo Nacional de Colombia, la Alcaldía Mayor de Bogotá con la Secretaría de Cultura, Recreación y Deporte y la Fundación Gilberto Alzate Avendaño ( FUGA), la Gobernación de Bolívar, el Instituto de Cultura y Turismo de Bolívar ICULTUR, el Museo Histórico de Cartagena de Indias MUHCA, la Conferencia Episcopal de Colombia, Fenalco Naranja, Mentes a la Carta, El Tiempo Casa Editorial, Servientrega, RTVC Señal Colombia y RTVC Señal Memoria. Así como las secretarías e institutos de Cultura y los principales museos del país.
Homenaje a Eduardo Butrón Hodwalker de Magangué, Bolívar
Este artista popular de Magangué, Bolívar, resultó ganador del primer premio del VI Salón BAT de Arte Popular con la obra Una mirada desde lo rural, de acuerdo con el jurado, por la transformación del espacio urbano de Magangué, un municipio que ha sido afectado por los problemas ambientales, el conflicto armado y la delincuencia.
Eduardo Butrón para crear sus obras utiliza desechos, como la basura que recoge del río Magdalena, dándole vida a sus creaciones, con un alto contenido ecológico y pedagógico, contribuyendo a la reconstrucción social. Refleja la problemática de su región y busca hacer un llamado a las autoridades y a la comunidad sobre la responsabilidad que tenemos todos los actores sociales en la preservación del medio ambiente. A través del mosaico, las instalaciones, los ensamblajes y del collage, refleja la identidad de la tierra que lo vio nacer, según el artista -“El río Magdalena representa una simbología que tiene la ciénaga, que forma parte de nuestra historia, de nuestra cultura, de la biodiversidad y de aquellos viajes en el David Arango, que nos identifica como seres ribereños”-.
Irradiación de valores ecológicos de los artistas populares
En medio de la variada topografía del país florece un conjunto de personas que han sido capaces de plasmar un sentido artístico personal, con mensajes de identidad. La mayoría de los artistas empíricos transforman desechos en arte y exploran con materiales que les da el entorno para crear. Sus obras las hacen con unas piedras, un bloque de arcilla, una goma elástica o diferenciadas fibras y, también con remanentes de papel, tela, alambre, plástico o pedazos de baldosas con diferenciados colores.
Esa producción exalta lo que somos como producto de nuestra historia, de las imágenes y recuerdos de la vida cotidiana, de las creencias, costumbres y personajes notables; todas son propuestas que enaltecen el carácter de la identidad nacional, de un medio ambiente natural, porque enseñan con ejemplo como su arte es sustentable.
Los artistas de todos los rincones de Colombia que tienen clara la importancia de la preservación del medio ambiente y la posibilidad de transformar materiales reciclados en arte popular están cordialmente invitados a participar en la convocatoria del VII Salón BAT de Arte Popular para que se expresen con el tema Colombia y el Medio Ambiente.
El VII Salón BAT de Arte Popular busca precisamente que todos los artistas tengan clara la importancia de la preservación del medio ambiente, de los ecosistemas y la posibilidad de encontrar en este espacio una plataforma de crecimiento y visibilidad para sus obras.
La convocatoria es abierta y de carácter nacional, y estará abierta desde el 14 de abril al 14 de julio de 2021, a través de la página web www.fundacionbat.com.co. Las exposiciones regionales de selección se llevarán a cabo entre el 2021 y 2022, el Gran Salón y el evento de premiación se realizarán en el 2022 y la itinerancia nacional tendrá lugar en los principales museos y centros culturales del país desde el 2022 hasta el 2024. Las exposiciones estarán acompañadas por actividades académicas y pedagógicas, producciones audiovisuales y editoriales.
Todos los artistas colombianos (inclusive los participantes y ganadores de las seis versiones anteriores), y los extranjeros residentes en el país, cuya formación profesional no haya sido en artes plásticas y similares, podrán participar en esta convocatoria con una obra que refleje el tema propuesto, Colombia y el Medio Ambiente.
A través del INPEC vamos a invitar a la población privada de la libertad a que participe, con los Programas de Desarrollo con Enfoque Territorial PDET se va a convocar a la población de dichos pueblos que se caracterizan por niveles de pobreza, en particular, de pobreza extrema y de necesidades insatisfechas, grado de afectación derivado del conflicto y presencia de cultivos de uso ilícito y de otras economías ilegítimas. Con la Conferencia Episcopal de Colombia llegaremos a las parroquias en los territorios y a los municipios a través de los medios comunitarios; con FONTUR promovemos la convocatoria en los 18 pueblos patrimonio, estaremos en todos los puntos de Servientrega del país, con las secretarías e institutos de cultura estaremos en todos los municipios de Colombia, con la Alcaldía de Bogotá en las 20 localidades y la comunidad de grafiteros. La convocatoria también se distribuirá a través de los museos aliados con el fin de tener un cubrimiento nacional.
Como novedad, junto con la Secretaría de Cultura, Recreación y Deporte de Bogotá se premiará al Arte Urbano Responsable, el ganador tendrá el privilegio de plasmar su mural en unos muros asignados por el Distrito en Bogotá. La maqueta que se presente debe estar diseñada teniendo en cuenta los dos muros que se especifican en la convocatoria, independientemente del lugar de proveniencia de la propuesta. El grafiti o la pintura mural ganadora será ejecutado por el artista durante fechas que coincidan con la realización del Gran Salón en Bogotá.
Este premio se crea con el ánimo de exaltar la estrategia implementada por la Alcaldía Mayor de Bogotá, a través de la Secretaría de Cultura, Recreación y Deporte, la cual genera inclusión y respeto por la diferencia y por nuestros entornos, al tiempo que se promueve el desarrollo de la libre expresión y se contribuye a la modificación de imaginarios, que han señalado el arte urbano como vandálico, transformando dichas percepciones para demostrar que el arte urbano es una práctica que pretende cambiar realidades y transformar argumentos con colores, creatividad y talento. Esta es una de las estrategias más significativas para la recuperación del espacio público, ya que genera acciones que impulsan el ejercicio de una ciudadanía activa, corresponsable y partícipe en la creación de la ciudad que todos soñamos.
Con qué técnicas pueden participar los artistas?
¿Qué premios se entregarán?
1. Un gran premio de $ 17.000.000 y estatuilla.
2. Tres primeros premios de $ 7.000.000 cada uno, con su respectiva estatuilla.
3. Dos segundos premios de $4.000.000 cada uno, con su respectiva estatuilla.
4. Un premio otorgado por el público de $4.000.000, con su respectiva estatuilla.
5.Un único premio de $10.000.000 y estatuilla para la mejor propuesta de Arte Urbano Responsable (pintura mural o grafiti).
6. Tantas menciones honoríficas como considere oportuno el jurado de premiación, cada una de las cuales se acompañará de una estatuilla.
7. Las obras premiadas pasarán a ser parte de la colección de arte popular de la Fundación BAT Colombia.
¿Cómo estará integrado el jurado?
La premiación se realizará en Bogotá con un jurado integrado por cinco miembros designados por el comité organizador del VII Salón BAT de Arte Popular, entre especialistas y artistas del arte popular, de amplia trayectoria y reconocimiento.
Para el caso del premio para la propuesta de Arte Urbano Responsable, hará parte del jurado un profesional especializado de la Secretaría Distrital de Cultura, Recreación y Deporte de Bogotá.
En las exposiciones regionales de selección el público hará parte del jurado, la obra con mayor votación será acreedora al premio del público.
Para mayor información visite: www.fundacionbat.com.co
Contacto de prensa: Luisa Venegas /Cel: 3014144339 /Luisa.venegas@secnewgate.co